Vue Js Remove Special Character from String:To remove special characters from a string in Vue.js, you can use a regular expression and the replace()
method.
First, create a regular expression that matches the special characters you want to remove. For example, if you want to remove all non-alphanumeric characters, you can use the expression /[^a-zA-Z0-9]/g
.
Next, use the replace()
method on the string you want to modify. Pass in the regular expression and a replacement string (in this case, an empty string ''
) as arguments.
How can special characters be removed from a string in Vue js?
This Vue.js code defines a simple UI with an input field that allows users to enter a string, and a computed property that updates the string by removing any special characters using regular expressions.
The regular expression /[^a-zA-Z0-9]/g
matches any character that is not a letter or a digit, and the g
flag indicates that the replacement should be global (i.e., applied to all instances of the pattern in the string).
The computed property updatedString
returns the result of calling the replace()
method on the myString
data property of the Vue instance, using the regular expression as the pattern and an empty string as the replacement.
Vue Js Remove Special Character from String Example
<div id="app">
<input type="text" v-model="myString" placeholder="Enter a string">
<p>{{ updatedString }}</p>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
myString: 'font-awesome-icons',
}
},
computed: {
updatedString() {
// remove special characters using regular expressions
return this.myString.replace(/[^a-zA-Z0-9]/g, '');
}
}
})
</script>
Output of Vue Js Remove Special Character from String
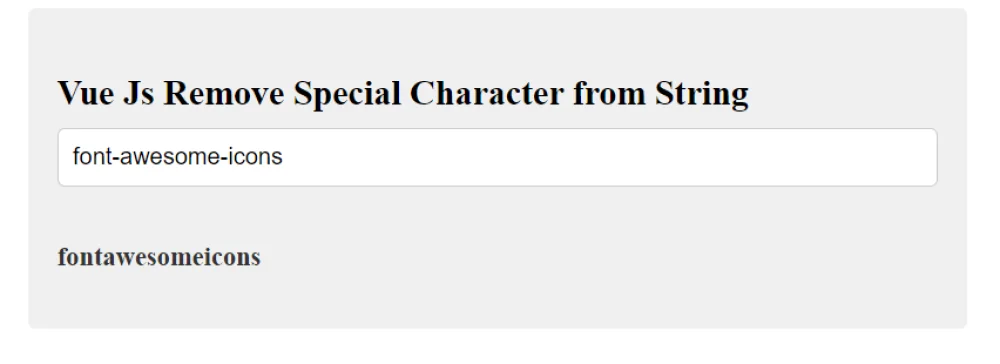